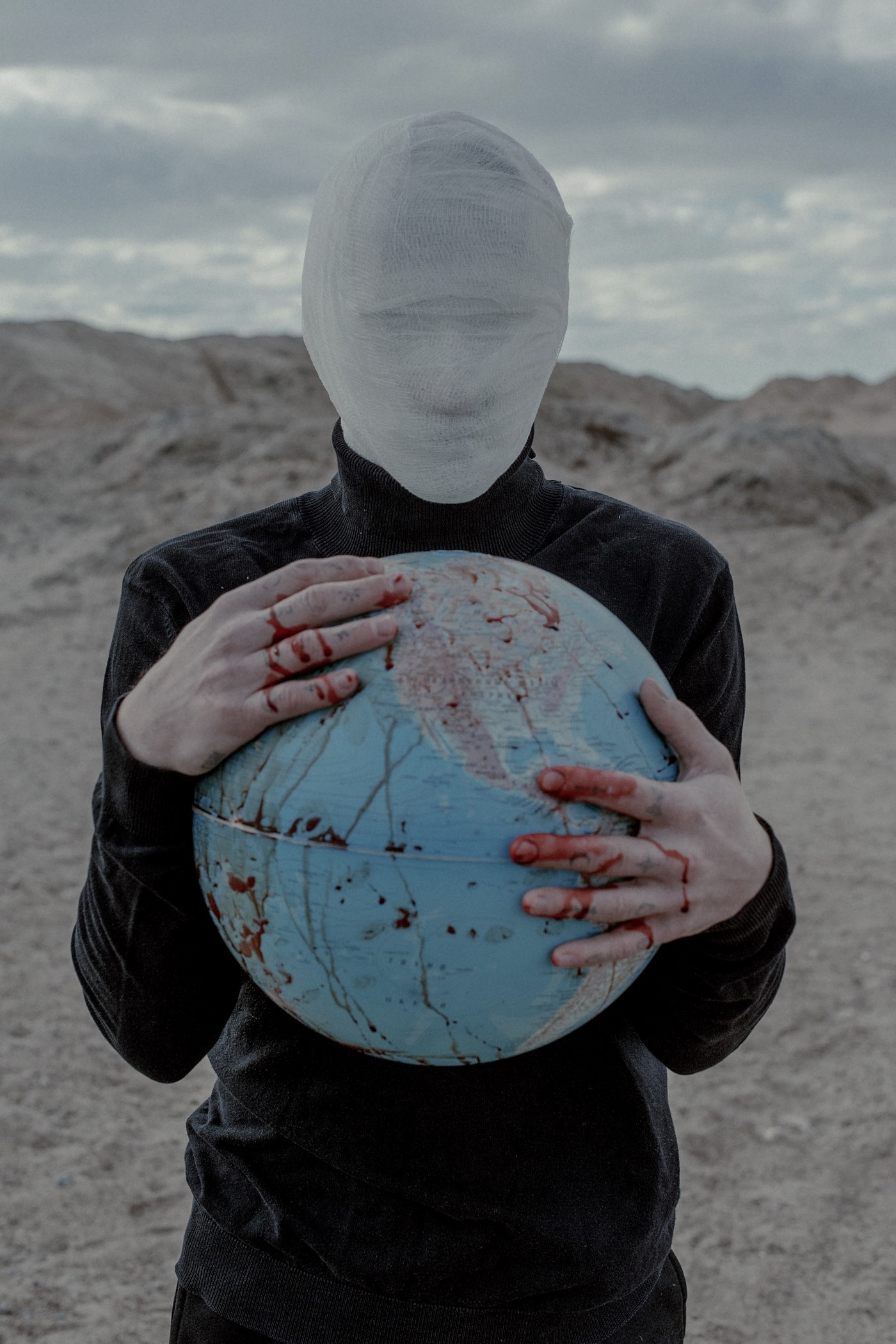
DNS Spoofing: Part Deux
I find this to be a bit of a double-edged sword. Yes, packet sniffing can be used maliciously. However, it can also be used to increase your situational awareness and add another layer to your cyber defense and response capabilities.
… and before moving on, this is illegal if you do this on a network on which you don’t have consent to gain access. Only do this on a virtual network that you have created, on devices you own, and on wi-fi you own.
Ok… let’s get on with it.
What are these packet sniffers? Simply put, they are a type of program that filter and read packets. These packets are packets of data being sent over a network between, for example, a laptop and a Wi-Fi router.
A program like this can be applied maliciously to gain other people’s data, or defensively to monitor for malicious packets.
Well funny you ask… I happen to have a python program to share with you that can sniff packets
Before I get into the code, allow me to provide some context and list out the environment set-up.
Furthermore, I know I mentioned that these packet sniffing programs can be used for malicious purposes. However, the particular packet sniffer that I’m going to be sharing is quite harmless. It can only sniff for http layers within a packet.
As a side note on HTTP, for the sake of digital hygiene, it is highly recommended to avoid inputting data on a website that is HTTP and not HTTPS. HTTPS websites have an added layer of security and therefore relatively safer than an HTTP website. Most professional websites run on the HTTPS protocol and therefore are not susceptible to the program I’m sharing in this post.
sudo
before calling the program from the terminal:Anyway, have fun. Here’s the code:
#!/usr/bin/env python
import scapy.all as scapy
from scapy.layers import http
def sniff(interface):
scapy.sniff(iface=interface, store=False, prn=processed_sniffed_packet)
def processed_sniffed_packet(packet):
if packet.haslayer(http.HTTPRequest):
url = packet[http.HTTPRequest].Host + packet[http.HTTPRequest].Path
print(url.decode())
sniff("eth0")
I’ll break down the code in the following paragraphs
So in a packet, there are different layers of data. To access these layers we need to recruit the aid of Scapy and instantiate its .sniff()
class. This is demonstrated below by highlighting the relevant lines in bold:
#!/usr/bin/env python
import scapy.all as scapy
from scapy.layers import http
def sniff(interface):
scapy.sniff(iface=interface, store=False, prn=processed_sniffed_packet)
def processed_sniffed_packet(packet):
if packet.haslayer(http.HTTPRequest):
url = packet[http.HTTPRequest].Host + packet[http.HTTPRequest].Path
print(url.decode())
sniff("eth0")
You also might have to pip install scapy
on your command line, as well.
Anyway, the function highlighted above in bold will catch the packets being sent over a network.
Now that we’ve “caught” a packet with the scapy.sniff()
class we can analyze and extract the contents. In this program, “to extract the contents” we are going to use a function that will filter out just the URLs of sites visited and then print them out. Just a reminder though, it will show URLs on the HTTP protocol, and not the HTTPS protocol.
Reference the lines of code in bold below:
#!/usr/bin/env python
import scapy.all as scapy
from scapy.layers import http
def sniff(interface):
scapy.sniff(iface=interface, store=False, prn=processed_sniffed_packet)
def processed_sniffed_packet(packet):
if packet.haslayer(http.HTTPRequest):
url = packet[http.HTTPRequest].Host + packet[http.HTTPRequest].Path
print(url.decode())
sniff("eth0")
As you can see above, we have an if statement
that filters out the layer in the packet that we want. It further filters out specific Fields within that layer in the following line. The data in those Fields will provide us the URL currently being viewed in the browser.
Below is a screenshot of side-by-side windows. The left window is the terminal in which I’m running the packet sniffing program. The window to the right shows the web browser from which the packets are being sniffed.
Thanks for reading and I hope you found this mildly entertaining.
If you enjoyed reading this article, support my writing by signing up for a Medium subscription.
Medium is a writing platform where writers from all over the world showcase their writing, covering any topic you can think of.
With a subscription you'll have unlimited access to all these writers and email notifications for my newly posted articles.
...but never fear, if you really enjoy my cybersecurity articles, then they will always be available here to read for free, for you!