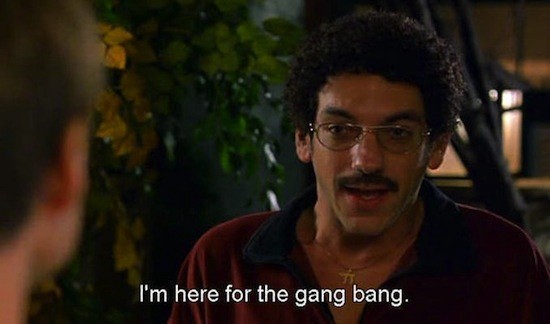
Man-in-the-Middle, ARP Spoofing???
This block of code can be broken down into four basic steps:
To read more about Scapy, check out their documentation HERE
import scapy.all as scpy
def scan(ip):
arp_request = scpy.ARP(pdst=ip)
broadcast = scpy.Ether(dst="ff:ff:ff:ff:ff:ff")
arp_request_broadcast = broadcast/arp_request
answered_list = scpy.srp(arp_request_broadcast, timeout = 1)[0]
for element in answered_list:
print(element[1].psrc)
print(element[1].hwsrc)
print("-----------------------------")scan("xxx.xxx.xxx.xxx/xxx")
That’s it. It’s a pretty short block of code. I’ll break it down by the variables used in the function.
This is just a place holder variable. You’ll have to know the ip address used by the devices on your network. I’ll explain how this is done below.
In the first variable created in the function, we instantiate an object using the scpy.ARP
class. This class creates an ARP packet and then we set the pdst
field to ip
We’ll instantiate another object with this variable. This time, the object we instantiate is an Ether frame in which we’ll wrap our ARP packet before sending. Also, the “ff:ff:ff:ff:ff:ff”
will serve as the destination MAC address for the response we receive after sending the ARP packet.
This variable bundles up the responses we get after sending the ARP packet so all the info we want is in one convenient location. Just use a forward slash (/) between the two variables to append them.
When we receive the response, it will come in the form of a list of two lists. This is because when we send our ARP packets, we’re going to send them to a series of IP address. We specify that series of IP addresses in the last line of the script when we call the function with a specified IP address in the parenthesis like this:
scan(“xxx.xxx.xxx.xxx/xxx”)
— except replace the Xs with numbers. To find out the IP addresses on your Wi-Fi network try the command route PRINT -4
(Windows) or route -n
(Linux/Apple) in your terminal/shell
To specify multiple IP addresses we’ll again use a forward slash ( / ). When we type scan(10.0.2.1/24)
for example, that tells the function to send an ARP packet to every IP address on the network starting with 10.0.2.1
through 10.0.2.24
. Some of those IP addresses won’t have a device associated to them and will not provide a response. Those will be considered ‘unaswered’ and all the IP addresses that send a response will be put in the answered_list
, which is the list that is returned at index 0.
The answered_list
is where we will parse out the info we want and see all the devices on the network.
This is the variable we use in the for loop
to parse out the pertinent info that we want. Basically, every item in the answered_list
will provide us info on every device on the network you’re scanning. The for loop
will iterate through each device and print the psrc
, which stands for packet source, a.k.a. the IP address of the device. It will also print the hwsrc
, which is the hardware source, a.k.a. the corresponding MAC address.
This function should be able to work on any OS because Scapy can be used on any OS. You just need to have python and then simply just download the scapy package.
Anyway, I hope you found this useful. You can copy the code. Refactor it. Make it your own. Share it. I don’t care. I just want to share it so more people can take control of their online security.
Thanks for reading.
If you enjoyed reading this article, support my writing by signing up for a Medium subscription.
Medium is a writing platform where writers from all over the world showcase their writing, covering any topic you can think of.
With a subscription you'll have unlimited access to all these writers and email notifications for my newly posted articles.
...but never fear, if you really enjoy my cybersecurity articles, then they will always be available here to read for free, for you!